Communicating down the hierarchy we have two ways to go:
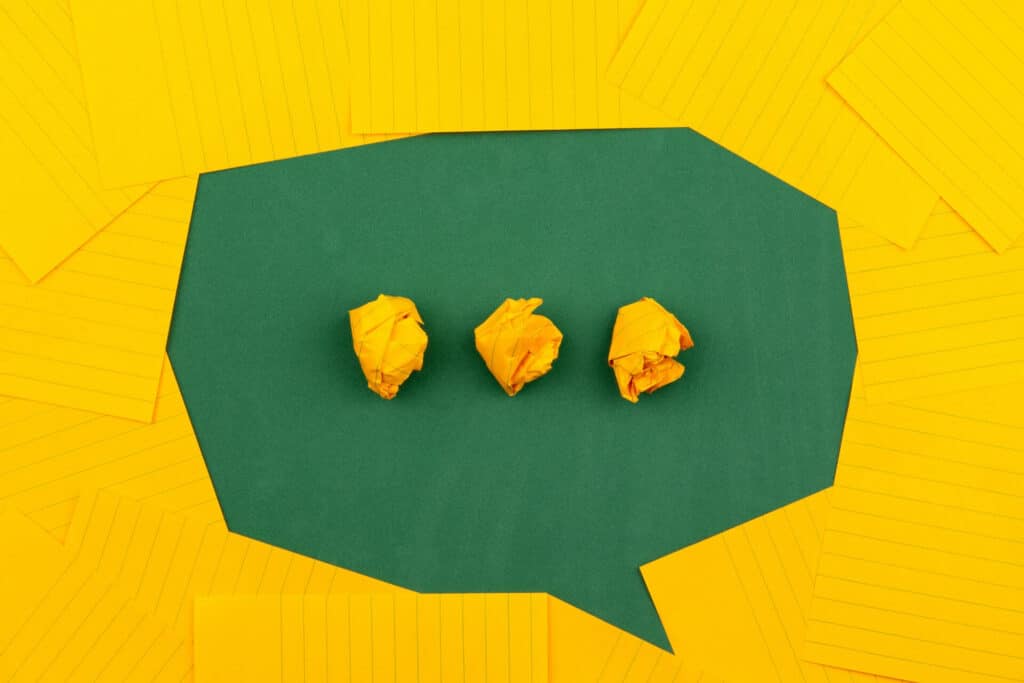
1. Using aura:attribute:
The aura: attribute is most commonly used to communicate from parent to child, kind of down the hierarchy. An attribute is more like a variable present on a component or event.
Parent Component.cmp (Sender)
<aura:component>
<c:ChildComponent Message="Hello" Message2="World"/>
</aura:component>
ChildComponent.cmp (Reciever)
<aura:attribute name="Message" type="String"/>
<aura:attribute name="Message2" type="String"/>
2. Using Aura : Method
We can use aura method tag to specify the method in the child component that we can call from the parent. In the parent component, we specify the child component and also impart an id to it. We can use this id to later refer and find the child component and then call to the aura method and attach parameters to be passed along.
ParentComponent.cmp(Sender)
<aura:component>
// put an id on the comp to find
<c:ChildComponent aura:id="compChild"/>
<lightning:button label="Just a button" onclick="{! c.onAction}" />
</aura:component>
ParentComponent.js
({
onAction : function(component, event, helper) {
var findcomp = component.find('compChild');
findcomp.someMethod("Param1", "Param2");
}
})
ChildComponent.cmp(Reciever)
<aura:component>
<aura:method name="someMethod" action="{!c.doAction}"
description="Sample method with parameters">
<aura:attribute name="param1" type="String" default="parameter 1"/>
<aura:attribute name="param2" type="Object" />
</aura:method>
</aura:component>
ChildComponent.js
({
doAction : function(cmp, event) {
var params = event.getParam('arguments');
if (params) {
var param1 = params.param1;
// add your code here
}
}
})
Read More: How to pass data from child to parent aura component
what is the use of your page if i am unable to copy the content of the page.
Awww… hey krishna
To avoid plagiarism right click is disabled buddy. Though you can always copy the code. On the code you find a copy button on right side. Use that. I guess it suffices ur need 😊
Hi,
Can you tell me the pros and cons of using both ways of parent to child communication. why do we need aura:method if we can just pass using attribute ?
thanks
In Salesforce, an aura method is a JavaScript function that you define inside a component. It helps you perform specific actions or handle events within that component.
While you can directly pass attributes in Salesforce components, using aura methods offers a more organized approach. Here’s why you might use aura methods:
Structure: Aura methods help you keep your component’s logic organized and separate from other components. It’s like having a dedicated set of instructions for that component.
Reusability: With aura methods, you can create functions that can be reused within the same component or even in other components. It saves you from rewriting similar code again and again.
Simplification: By breaking down complex functionality into smaller methods, aura methods make your code easier to understand and manage. Each method can handle a specific task, making the overall code more modular.
Event handling: Aura methods are particularly useful when it comes to handling events, such as button clicks or data changes. You can define a method to respond to those events and perform the desired actions.
In summary, aura methods provide a structured way to handle interactions within Salesforce components, make your code more reusable and maintainable, and simplify event handling
Thank you for sharing this in such a neat manner 🙂