In this post, we are going to create a very basic dynamic table to fetch the latest files from a list of files with the same name. We are thereby creating a custom table on the record page to pull the files related to that record dynamically with a click of a button. To have it on a quick access button, we are adding this LWC component to an aura application. Without further ado, let’s get started.
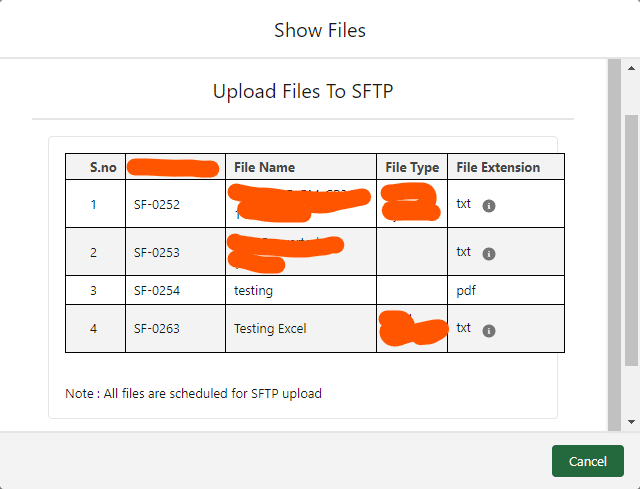
We have three basic HTML, JS, and MetaXML to set up in the Lightning Component Bundle for this requirement.
SFDX:Lightning Web Component >> New >>
filesTable.html
<template>
<div class="slds-box slds-theme–default">
<table class="slds-table slds-table_cell-buffer slds-table_bordered slds-table_striped">
<thead>
<tr class="slds-line-height_reset">
<th class="" scope="col">
S.no
</th>
<th class="" scope="col">
Spec File
</th>
<th class="" scope="col">
File Name
</th>
<th class="" scope="col">
File Type
</th>
<th class="" scope="col">
File Extension
</th>
</tr>
</thead>
<tbody>
<template iterator:it={filetabledata}>
<tr class="slds-hint-parent" key = {it.value.sno}>
<td data-label="Spec File">
<div class="slds-cell-wrap">{it.value.sno}</div>
</td>
<td data-label="Spec File">
<div class="slds-cell-wrap">{it.value.file}</div>
</td>
<td data-label= "File Name">
<div class="slds-cell-wrap">{it.value.name}</div>
</td>
<td data-label="File Type">
<div class="slds-cell-wrap">{it.value.type}</div>
</td>
<td data-label="File Extension">
<div class="slds-cell-wrap">{it.value.ext}</div>
</td>
</tr>
</template>
</tbody>
</table>
<div class="slds-p-top_x-small">Note : All files are scheduled for SFTP upload</div>
</div>
</template>
filesTable.js
import { LightningElement,wire,api,track } from 'lwc';
import fetchfiles from '@salesforce/apex/fileTable.fetchfiles';
export default class FilesTable extends LightningElement {
@track filetabledata=[];
@api recordId;
records;
i=0;
get count(){
return `${this.i}`;
}
@wire(fetchfiles,{
recordId:'$recordId'
})
wiredclass({ error, data }) {
//*********** we are here manipulating data before sending it to html, to make sequence and show xlsx files as text*****//
if (data) {
this.tableDataTmp = data;
console.log(JSON.stringify(data));
let index = 0;
this.tableData = data.map( item =>{
let extension = item['File_Extension__c'] == 'xlsx'?'txt':item['File_Extension__c'];
index += 1;
var temData = {
'sno':index,
'ext':extension,
'name':item['File_Name2__c'],
'file': item['Name'],
'type': item['File_Type__c']
}
this.filetabledata.push(temData);
return{...item,'File_Extension__c':extension}
})
} else if ( error ) {
this.records = undefined;
}
}
}
filesTable.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<isExposed>false</isExposed>
<targets>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>ScreenAction</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
Apex
public with sharing class fileTable {
public fileTable() {}
@AuraEnabled( cacheable = true )
public static List<File__c> fetchfiles(id recordId) {
Map<string,File__c> filemp = New Map<string,File__c>();
List <Spec__c> speclist = [Select id,(SELECT Id, Name,File_Extension__c, File_Name2__c, File_Type__c FROM Spec_Files__r Order by File_Name2__c,CreatedDate ASC) from Spec__c where id=:recordId];
for(Spec__c spec : speclist){
for(File__c f : spec.Spec_Files__r){
filemp.put(f.File_Name2__c,f);
}
}
return filemp.values();
}
}
AURA App: Passing the record Id to the LWC component
<aura:component implements="force:lightningQuickAction,force:hasRecordId">
<aura:attribute name="recordId" type="String" />
<c:filesTable recordId="{!v.recordId}" />
</aura:component>
There ain’t much to explain here. We implemented the ‘force:hasRecordId’ to get the recordId and add ‘force:lightningQuickAction’ to add the component on quick action.
Read More: Create a contact form in LWC
Hope You Have An Amazing Day! Let me know in the comments if it helped you learn something!